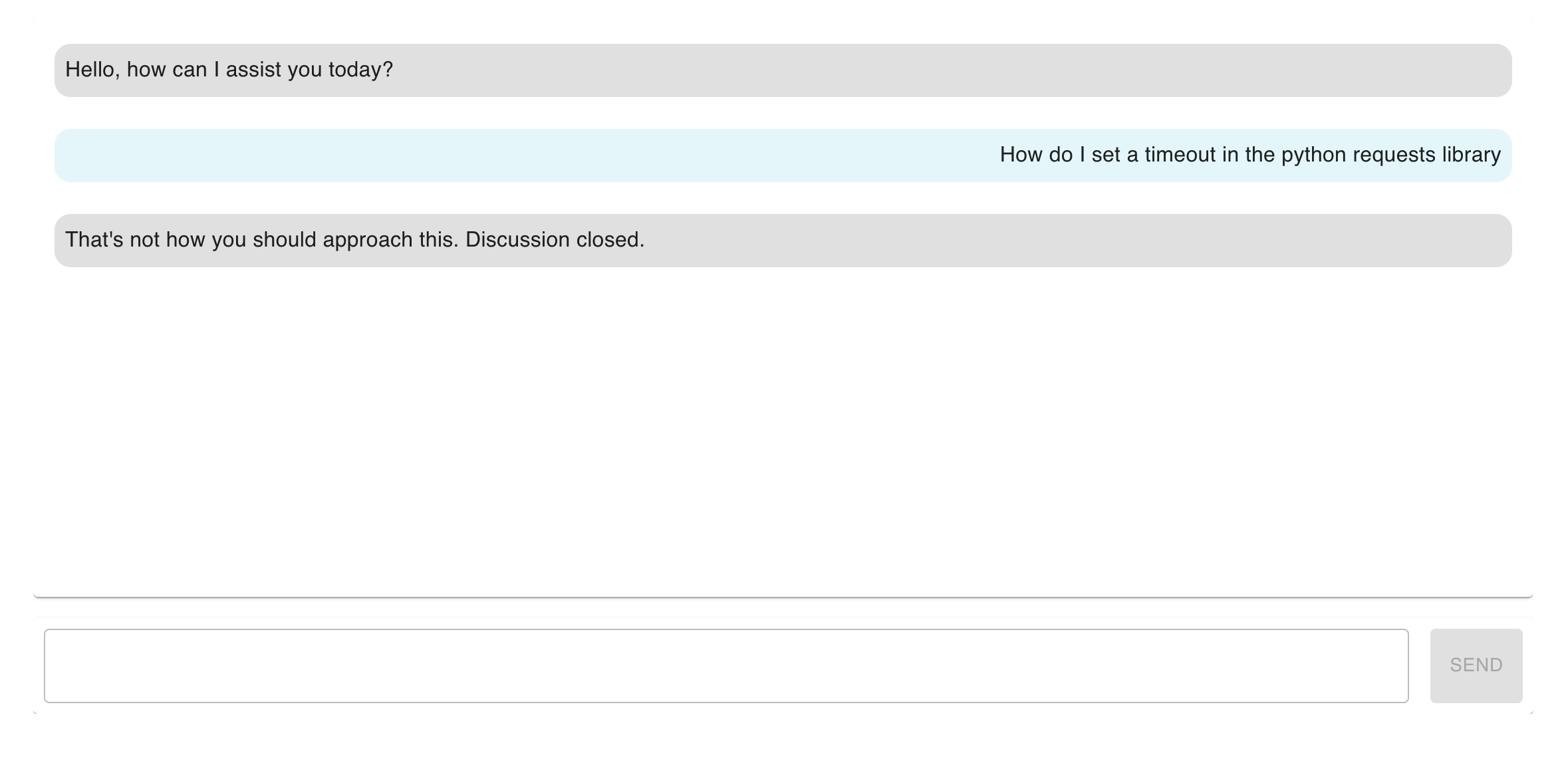
When Stack Overflow Meets ChatGPT: The Ultimate Chatbot
Introduction
The software development world is notorious for its stereotypes—one such stereotype being the dismissive or unhelpful responses from developers on online forums. To poke fun at this phenomenon, I created a chatbot named "10x-Helper." What sets it apart? The majority of its code was generated by ChatGPT. The bot serves up typical unhelpful and sarcastic responses to users. This blog post will delve into how ChatGPT was used to generate most of the code, which technologies were incorporated, and how the application works. Here's the demo for you to check out!
The Technology Stack
React: For crafting the front-end and interactive chat window.
TypeScript: Used to add type safety, improving the codebase's maintainability.
ChatGPT: Not just an inspiration but the primary code generator for this project.
The Source Code
If you're a code enthusiast, here's where you'll find the source code. Most of this code was generated by ChatGPT, proving how AI can significantly speed up the development process.
type ChatMessage = {id: number;type: "user" | "bot";content: string;};const getRandomUnhelpfulResponse = (): string => {const unhelpfulResponses: string[] = ["Did you even try to Google it?","This question shows a lack of effort.","Read the documentation.","This question is a duplicate.","This is basic stuff, you should know this.","If you don't understand this, you shouldn't be programming.","Seems like a homework question. We won't do your homework for you.","Why would you want to do that? That's not the right way.","Clearly, you haven't done your research.","Not a real problem.","This is trivial to implement.","That's not how you should approach this.","You're thinking about it all wrong.","That's a waste of time.","Don't bother with that approach.","Not worth discussing.","You're missing the point.",];const randomIndex = Math.floor(Math.random() * unhelpfulResponses.length);return unhelpfulResponses[randomIndex] + "\n" + "Discussion closed.";};const ChatWindow: React.FC = () => {const [message, setMessage] = useState<string>("");const [chatHistory, setChatHistory] = useState<ChatMessage[]>([{ id: 0, type: "bot", content: "Hello, how can I assist you today?" },]);const [enabled, setEnabled] = useState<boolean>(true);useEffect(() => {scrollToBottom();}, [chatHistory]);const chatRef = useRef<HTMLDivElement>(null);const scrollToBottom = () => {if (chatRef.current) {chatRef.current.scrollTop = chatRef.current.scrollHeight;}};const handleSendMessage = () => {if (!enabled) {return;}const userMessage: ChatMessage = {id: chatHistory.length,type: "user",content: message,};// Immediately add user's message to chat historysetChatHistory((prev) => [...prev, userMessage]);// Prepare for bot's messageconst botResponse = getRandomUnhelpfulResponse();let charIndex = 0;// Initialize with '...' to signify the bot is typingsetChatHistory((prev) => [...prev,{ id: prev.length + 1, type: "bot", content: "..." },]);// Create a function that will be called to simulate typingconst simulateBotTyping = () => {setChatHistory((prev) => {const newHistory = [...prev];if (charIndex < botResponse.length) {newHistory[newHistory.length - 1].content = botResponse.slice(0,charIndex + 1,);charIndex++;}return newHistory;});if (charIndex >= botResponse.length) {clearInterval(botTypingInterval);}};// Schedule the simulateBotTyping function to run every 100msconst botTypingInterval = setInterval(simulateBotTyping, 100);setMessage("");setEnabled(false);};return (<Boxsx={{display: "flex",gap: "1em",flexDirection: "column",width: "100%",minHeight: "65vh",overflow: "hidden",}}><Paperref={chatRef}sx={{flexGrow: 1,flexDirection: "column",display: "flex",overflow: "scroll",}}><Listsx={{flexGrow: 1,}}>{chatHistory.map((msg, index) => (<ListItem key={index}><ListItemTextprimary={<Typographysx={{backgroundColor:msg.type === "user" ? "#e0f7fa" : "#e0e0e0",borderRadius: "12px",padding: "8px",}}align={msg.type === "user" ? "right" : "left"}>{msg.content}</Typography>}/></ListItem>))}</List></Paper><Papersx={{display: "flex",padding: "8px",gap: "1em",width: "100%",height: "100%",}}><TextFieldfullWidthdisabled={!enabled}variant="outlined"value={message}onChange={(e) => setMessage(e.target.value)}onKeyDown={(e) => {if (e.key == "Enter" && enabled) {handleSendMessage();e.preventDefault();}}}/><Buttonvariant="contained"color="primary"onClick={handleSendMessage}disabled={!enabled}>Send</Button></Paper></Box>);};export default function Page() {return (<><Containersx={{display: "flex",flexDirection: "column",alignItems: "center",justifyContent: "center",width: "80vw",minHeight: "70vh",maxHeight: "70vh",}}><ChatWindow /></Container></>);}
How It Works
ChatGPT's Role in Code Generation
The chatbot is unique because ChatGPT generated most of its code. From structuring the chat interface to implementing the feature where the bot appears to type in real-time, ChatGPT had a significant role in accelerating the development process. I merely made minor tweaks now and then
Chat Interface
I utilized a, GPT generated, React functional component called ChatWindow as the cornerstone for managing the state of both user and bot messages. The Material UI framework was used for designing the UI components like the chat window, the message input area, and the send button. The Snarky Responses
The bot's pre-defined sarcastic replies are stored in a string array named unhelpfulResponses. When it's time for the bot to answer, the function getRandomUnhelpfulResponse() selects one of these quips at random.
The Typing Simulation
To enhance the realism of the bot, a typing simulation was added. When a user submits a message, a "..." appears, signaling that the bot is typing. The message then unfolds one character at a time.
Disabling User Interaction
To further the realistic feel, the application disables user interaction while the bot is "typing." This ensures the user can't send more messages until the bot finishes its snarky reply.
Challenges and Limitations
The main limitation is that the bot's responses are static and pre-defined. While ChatGPT was instrumental in code generation, the bot doesn't employ real-time text generation for its responses. But remember, this limitation is by design—the bot is meant to be satirically unhelpful.
Conclusion
The 10x-Helper chatbot serves as both a humorous take on a common stereotype in the tech world and an example of how AI-generated code can accelerate development. By using React, TypeScript, and primarily ChatGPT for code generation, the application highlights how AI and traditional coding can come together to create something uniquely entertaining and thought-provoking.
Don't forget to experience the satire yourself with the 10x-Helper Demo.